How to use
Import the client from node modules:
import Autograph from 'autograph-client'
Create an instance of the client class:
const autograph = new Autograph()
We do not pass any config to it yet.
Config
You will need to pass this object to render
method of an instance.
Let's take a closer look at the details of what it consists of.
apiKey
Your Autograph API key. Required.
appMode
Which mode should Autograph be loaded in. Required.
prepareDocument
, prepareDocumentWithTemplate
, createTemplate
, sign
.
contacts
Array of objects of the following structure:
{
name: 'John Doe',
email: 'john@doe.com'
}
This contacts list will be automatically added to the recipients list of a signature request. Optional.
containerElement
HTML container element, client web component will be rendered inside it. Required.
fileUploadServices
Optional object, containing configs for each external file storage service, you'd want a user to be able to upload a file from, currently Google Drive and Dropbox are supported. More on this here.
getTemplatesFunction
Optional. Provide an async function, which fetches the list of templates. The function must return a promise, that resolves with an array of template objects, the following fields are mandatory for each of the objects: createdAt
, name
, templateId
- each is a string. The Autograph client will check if the function is present in the config object, render Choose from Templates button accordingly and call the function when the button is clicked.
The function will be called with the only argument - template type string (TemplateType).
Choose from Templates button will be rendered only if appMode
is prepareDocument
.
Please make sure the function returns a promise, not the data, and each object in an array has mandatory fields.
getContactsFunction
Optional. Provide an async function, which fetches contacts, to show autocomplete options on the "Add recipients" step, when entering contact's name. The function must return a promise, that resolves with an array of contact objects, the following fields are mandatory for each of the objects: id
, name
, email
- each is a string. The function will be called with the only argument - string value of name input field.
Please make sure the function returns a promise, not the data, and each object in an array has mandatory fields.
existingSignatureRequestId
ID of existing signature request, to be combined with prepareDocument
app mode, to reuse existing signature request and skip selecting documents. Optional.
existingSignatureTemplateId
ID of existing signature template, to be combined with createTemplate
app mode, to reuse existing signature template and skip selecting documents. Optional.
signatureRequestType
A string representing signature request type, possible values:
SEQUENTIAL
, BULK
, COLLABORATIVE
Required only if app mode is prepareDocumentWithTemplate
.
signFileId
ID of a file, which needs to be signed. Required only when using sign
app mode.
templateId
ID of a template, to use when preparing a document using a template. Required only when using prepareDocumentWithTemplate
app mode.
locale
A string, representing locale name, that will be used for all text elements within the client. Currently we support only limited number of locales. If unsupported locale will be passed it will fall back to en-US
.
en-US
, es-LA
, fr-CA
, pt-BR
theme
Optional object, containing properties for theming. Currently, only primaryColor
property is supported. It may contain a string representing any color in any form, that can be put into CSS variable: color name, hex
, rgb
, hsl
and so on. Defaults to #2265D2
. For example this setting:
{
theme: {
primaryColor: 'darkgreen'
}
// ...rest of config properties
}
will lead to this result:
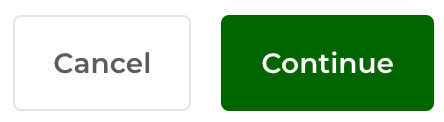
token
JWT of a user on whose behalf all actions inside Autograph will be done. Required.
inPersonEmail and inPersonToken
Strings representing an email and a token of a user that will be used in the in-person signing mode, in that case required.
Instance methods
render
To render the client inside a container, please call render
method of the client instance:
autograph.render(config)
This will create client's web component HTML element and will put it in DOM.
destroy
To unmount the client please use destroy
instance method:
autograph.destroy()
This will remove client element from DOM tree and do some internal stuff. You should use this method every time before re-rendering client with new config.
on
For subscribing to client events you should use on
instance method:
autograph.on(eventName, callback)
Events
For details about every event and its payload please refer to Events page.